背景
因业务需求,需要与其他平台实现融合登录,根据提供融合登录方的文档内容,对AES
加密解密相关内容使用.NET CORE
来进行一次实现。以下记录实现AES
加解密后的内容,便以后备用。
AES
高级加密标准(英语:Advanced Encryption Standard
,缩写:AES
),在密码学中又称Rijndael
加密法,是美国联邦政府采用的一种区块加密标准。这个标准用来替代原先的DES
,已经被多方分析且广为全世界所使用。严格地说,AES
和Rijndael
加密法并不完全一样(虽然在实际应用中二者可以互换),因为Rijndael
加密法可以支持更大范围的区块和密钥长度:AES
的区块长度固定为128
比特,密钥长度则可以是128
、192
或256
比特;而Rijndael
使用的密钥和区块长度可以是32
位的整数倍,以128
位为下限,256
比特为上限。包括AES-ECB
、AES-CBC
、AES-CTR
、AES-OFB
、AES-CFB
.NET CORE 的AES加解密实现
public enum CiphertextType
{
/// <summary>
/// Base64
/// </summary>
Base64 = 1,
/// <summary>
/// 16进制字符串
/// </summary>
Hex = 2
}
public static class EncryptionAes
{
#region AES加密
/// <summary>
/// AES加密
/// </summary>
/// <param name="source"></param>
/// <param name="key">密钥</param>
/// <param name="iv">初始向量</param>
/// <param name="padding">填充模式</param>
/// <param name="mode">加密模式</param>
/// <param name="ciphertextType">密文类型</param>
/// <returns></returns>
public static (bool isSuccess, string text) AESEncrypt(this string source, string key, string iv = "", PaddingMode padding = PaddingMode.PKCS7, CipherMode mode = CipherMode.CBC, CiphertextType ciphertextType = CiphertextType.Base64)
{
try
{
byte[] keyBytes = Encoding.UTF8.GetBytes(key);
byte[] textBytes = Encoding.UTF8.GetBytes(source);
byte[] ivBytes = Encoding.UTF8.GetBytes(iv);
byte[] useKeyBytes = new byte[16];
byte[] useIvBytes = new byte[16];
if (keyBytes.Length > useKeyBytes.Length)
{
Array.Copy(keyBytes, useKeyBytes, useKeyBytes.Length);
}
else
{
Array.Copy(keyBytes, useKeyBytes, keyBytes.Length);
}
if (ivBytes.Length > useIvBytes.Length)
{
Array.Copy(ivBytes, useIvBytes, useIvBytes.Length);
}
else
{
Array.Copy(ivBytes, useIvBytes, ivBytes.Length);
}
Aes aes = System.Security.Cryptography.Aes.Create();
aes.KeySize = 256;//秘钥的大小,以位为单位,128,256等
aes.BlockSize = 128;//支持的块大小
aes.Padding = padding;//填充模式
aes.Mode = mode;
aes.Key = useKeyBytes;
aes.IV = useIvBytes;//初始化向量,如果没有设置默认的16个0
ICryptoTransform cryptoTransform = aes.CreateEncryptor();
byte[] resultBytes = cryptoTransform.TransformFinalBlock(textBytes, 0, textBytes.Length);
return (true, CipherByteArrayToString(resultBytes, ciphertextType));
}
catch (Exception ex)
{
return (false, ex.Message);
}
}
#endregion
#region AES解密
/// <summary>
/// AES解密
/// </summary>
/// <param name="source"></param>
/// <param name="key">密钥</param>
/// <param name="iv">初始向量</param>
/// <param name="padding">填充模式</param>
/// <param name="mode">加密模式</param>
/// <param name="ciphertextType">密文类型</param>
/// <returns></returns>
public static (bool isSuccess, string text) AESDecrypt(this string source, string key, string iv = "", PaddingMode padding = PaddingMode.PKCS7, CipherMode mode = CipherMode.CBC, CiphertextType ciphertextType = CiphertextType.Base64)
{
try
{
byte[] keyBytes = Encoding.UTF8.GetBytes(key);
byte[] textBytes = CiphertextStringToByteArray(source, ciphertextType);
byte[] ivBytes = Encoding.UTF8.GetBytes(iv);
byte[] useKeyBytes = new byte[16];
byte[] useIvBytes = new byte[16];
if (keyBytes.Length > useKeyBytes.Length)
{
Array.Copy(keyBytes, useKeyBytes, useKeyBytes.Length);
}
else
{
Array.Copy(keyBytes, useKeyBytes, keyBytes.Length);
}
if (ivBytes.Length > useIvBytes.Length)
{
Array.Copy(ivBytes, useIvBytes, useIvBytes.Length);
}
else
{
Array.Copy(ivBytes, useIvBytes, ivBytes.Length);
}
Aes aes = System.Security.Cryptography.Aes.Create();
aes.KeySize = 256;//秘钥的大小,以位为单位,128,256等
aes.BlockSize = 128;//支持的块大小
aes.Padding = padding;//填充模式
aes.Mode = mode;
aes.Key = useKeyBytes;
aes.IV = useIvBytes;//初始化向量,如果没有设置默认的16个0
ICryptoTransform decryptoTransform = aes.CreateDecryptor();
byte[] resultBytes = decryptoTransform.TransformFinalBlock(textBytes, 0, textBytes.Length);
return (true, Encoding.UTF8.GetString(resultBytes));
}
catch (Exception ex)
{
return (false, ex.Message);
}
}
/// <summary>
/// 通过密文返回加密byte数组
/// </summary>
/// <param name="ciphertext"></param>
/// <param name="ciphertextType"></param>
/// <returns></returns>
public static byte[] CiphertextStringToByteArray(string ciphertext, CiphertextType ciphertextType)
{
byte[] cipherByte;
switch (ciphertextType)
{
case CiphertextType.Base64:
cipherByte = Convert.FromBase64String(ciphertext);
break;
case CiphertextType.Hex:
cipherByte = HexStringToByteArray(ciphertext);
break;
default:
cipherByte = Convert.FromBase64String(ciphertext);
break;
}
return cipherByte;
}
/// <summary>
/// 通过加密的Byte数组返回加密后的密文
/// </summary>
/// <param name="cipherByte"></param>
/// <param name="ciphertextType"></param>
/// <returns></returns>
public static string CipherByteArrayToString(byte[] cipherByte, CiphertextType ciphertextType)
{
string ciphertext = "";
switch (ciphertextType)
{
case CiphertextType.Base64:
ciphertext = Convert.ToBase64String(cipherByte);
break;
case CiphertextType.Hex:
ciphertext = ByteArrayToHexString(cipherByte);
break;
default:
ciphertext = Convert.ToBase64String(cipherByte);
break;
}
return ciphertext;
}
/// <summary>
/// Hex字符串转Byte
/// </summary>
/// <param name="hexContent"></param>
/// <returns></returns>
public static byte[] HexStringToByteArray(string hexContent)
{
hexContent = hexContent.Replace(" ", "");
byte[] buffer = new byte[hexContent.Length / 2];
for (int i = 0; i < hexContent.Length; i += 2)
{
buffer[i / 2] = (byte)Convert.ToByte(hexContent.Substring(i, 2), 16);
}
return buffer;
}
/// <summary>
/// Byte转Hex字符串
/// </summary>
/// <param name="data"></param>
/// <returns></returns>
public static string ByteArrayToHexString(byte[] data)
{
StringBuilder sb = new StringBuilder(data.Length * 3);
foreach (byte b in data)
{
sb.Append(Convert.ToString(b, 16).PadLeft(2, '0'));
}
return sb.ToString().ToUpper();
}
#endregion
}
调用AES加解密
class Program
{
static void Main(string[] args)
{
var ddd = "111111111" .AESEncrypt("3EDsw9[A4W5FdS{]3W","0102030504030201", PaddingMode.PKCS7);
Console.WriteLine($"加密:{ddd.text}");
Console.WriteLine($"解密:{(ddd.text.AESDecrypt("3EDsw9[A4W5FdS{]3W", "0102030504030201", PaddingMode.PKCS7)).text}");
Console.Read();
}
}
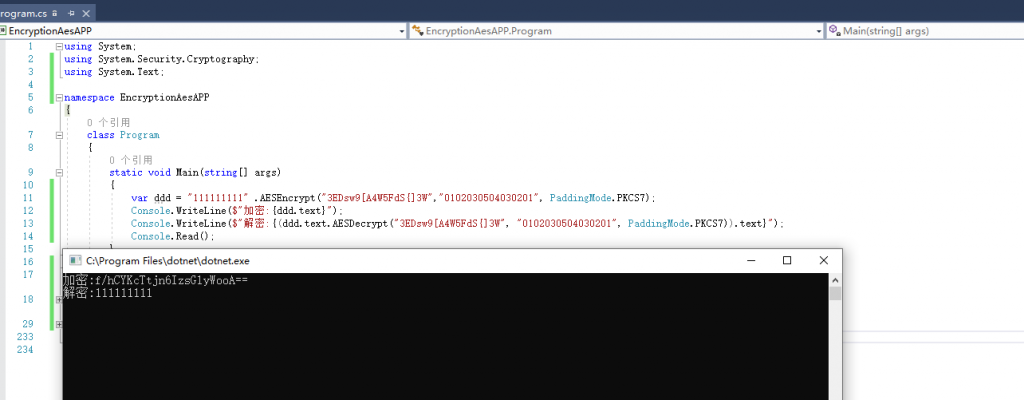
示例下载
.Net Core实现AES加解密示例
转载请注明:清风亦平凡 » .Net Core实现AES加解密